If you are a mobile application developer. You definitely have seen a blank white screen before application first screen launch.
Mobile applications do take some amount of time to start up, especially on a cold start. There is a delay there that you may not be able to avoid. This is an unconfigured section of the app that first time they launch. Instead of leaving a blank screen during this time. We should use it to display a splash screen until the app takes to configure itself.
In a flutter application, you might have observed that a long blank screen. Actually, there is a Splash screen, but it is with a white background and nobody can understand that there is already a splash screen for iOS and Android by default.
This is actually cool because the only thing that the developer needs to do is to put the branding image in the right place and the splash screen will start working just like that.
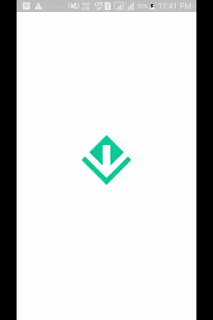
You can follow these steps to set up launch images in Android and iOS respectively.
For the Android platform.
1. Find the "android" folder in your Flutter project and browse to app -> src -> main -> res folder and place all of the variants of your branding image in the corresponding folders. For example:
3. If you want to change the application icon. Go to the app -> src -> main-> manifest file and declare application icon there as we did below.
For the iOS platform.
1. Find the "ios" folder in your Flutter project and go to Runner -> Assets.xcassets -> LaunchImage.imageset. There should be LaunchImage.png, LaunchImage@2x.png etc. Now you have to replace these images with you branding image variants. For example:
If LaunchImage@4x.png does not exist by default. You can easily create it and you have to declare it in the Contents.json file as well, which is in the same directory as the images. After the change Contents.json file looks like this :
Now, run your app and you will see. On the Android and iOS, you should have the right Splash Screen with the branding image that you added and the blank white screen removed as you can see above.
Then in your MyApp(), in your initState(), you can use Future.delayed to set up a timer or call any API. Until the response is returned from the Future, your launch icons will be shown and then as the response comes, you can move to the screen you want to go to after the splash screen.
We hope the post helped you to learn about default blank screen in Flutter Application and you able to kill blank screen before app configures itself. If you facing any issue please feel free to ask from the comment section below.
Mobile applications do take some amount of time to start up, especially on a cold start. There is a delay there that you may not be able to avoid. This is an unconfigured section of the app that first time they launch. Instead of leaving a blank screen during this time. We should use it to display a splash screen until the app takes to configure itself.
In a flutter application, you might have observed that a long blank screen. Actually, there is a Splash screen, but it is with a white background and nobody can understand that there is already a splash screen for iOS and Android by default.
This is actually cool because the only thing that the developer needs to do is to put the branding image in the right place and the splash screen will start working just like that.
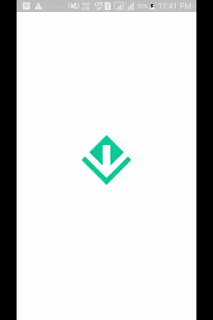
Before After
For the Android platform.
1. Find the "android" folder in your Flutter project and browse to app -> src -> main -> res folder and place all of the variants of your branding image in the corresponding folders. For example:
- The image with density 1 needs to be placed in mipmap-mdpi.
- The image with density 1.5 needs to be placed in mipmap-hdpi.
- The image with density 2 needs to be placed in mipmap-xdpi.
- The image with density 3 needs to be placed in mipmap-xxdpi.
- The image with density 4 needs to be placed in mipmap-xxxdpi.
By default for the android folder there isn't drawable-mdpi, drawable-hdpi etc. but you can create if he wants to put all variants there.
2. After that, go to app -> src -> main -> res-> drawable and open launch_background.xml. Inside this file, you will see why the splash screen background is white. To apply the branding image we need to change it as below. We have to uncomment some of the XML code in your launch_background.xml file.3. If you want to change the application icon. Go to the app -> src -> main-> manifest file and declare application icon there as we did below.
For the iOS platform.
1. Find the "ios" folder in your Flutter project and go to Runner -> Assets.xcassets -> LaunchImage.imageset. There should be LaunchImage.png, LaunchImage@2x.png etc. Now you have to replace these images with you branding image variants. For example:
- The image with density 1 needs to override LaunchImage.png,
- The image with density 2 needs to override LaunchImage@2x.png,
- The image with density 3 needs to override LaunchImage@3x.png,
- The image with density 4 needs to override LaunchImage@4x.png,
If LaunchImage@4x.png does not exist by default. You can easily create it and you have to declare it in the Contents.json file as well, which is in the same directory as the images. After the change Contents.json file looks like this :
Now, run your app and you will see. On the Android and iOS, you should have the right Splash Screen with the branding image that you added and the blank white screen removed as you can see above.
Then in your MyApp(), in your initState(), you can use Future.delayed to set up a timer or call any API. Until the response is returned from the Future, your launch icons will be shown and then as the response comes, you can move to the screen you want to go to after the splash screen.
We hope the post helped you to learn about default blank screen in Flutter Application and you able to kill blank screen before app configures itself. If you facing any issue please feel free to ask from the comment section below.