To get dynamic data in a mobile application. We need to connect with a server to get and post some data. To achieve it, we always use HTTP to perform curl requests and create a connection between applications and server at a certain period to send or receive the data request from an application to the server.
Flutter providing us http to connect a mobile application with a server to perform GET, POST and other requests. POST and GET are two most commonly used HTTP methods for request and response between the client and the server. GET method basically requests data from a specified resource, whereas Post method submits data to be processed to a specified resource.
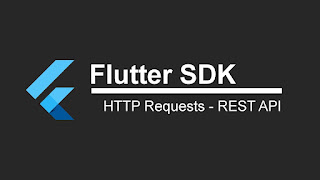
If you are looking some advance http client to handle interceptors, logs, cache etc. Then you should try Dio http client that we have explained here: Flutter - Dio client to create Http Request
In this post, we are going to learn about HTTP/HTTPS POST & GET Requests. We'll see, how can get data from the server with the help of a utility class that we have created to perform GET and POST in this sample. In this example, we'll get movie list from themoviedb. We have generated a testing key from here. To run the following sample we have to create it. So create it before start development. The final output of this post example will look like below. When you'll create project with the help of following steps.
Let's start the development of this sample with the help of following steps:
Creating a new Project
1. Create a new project from File ⇒ New Flutter Project with your development IDE.
2. Open pubspec.yaml file and add following dependancies
http: ^0.12.0
transparent_image: ^0.1.0
3. After that open main.dart file and edit it. As we have set our theme and change debug banner property of Application. This is our main widget of the example. Here, we have created a grid list widget to display a list of search movies and we have created an instance of MovieApi() to get a list of items from the server.
4. In the following file, we have created a method to access our network layer method. As you can see, we using a POST method to push a query to server and parsing response with the help of base model class.
5. Now create basemodel.dart file. As we said, we use this class to create an object from the server response.
6. Now create PODA class to keep fields of a movie.
7. Here, we have our main file of this post that we were talking about. This is a network util class that we can use it anywhere to perform http request in a Flutter Application. In the following class, we have created a method for GET and POST request. You can add other methods of http according to your requirement. In this file, we have created a field BASE_URL. It contains our base address of the server. We can append a method of server function. Like in this example, we use a search method of themoviedb to get a search result. If you going to build a big application. Then you should use this pattern to perform the server task.
If you have followed the article carefully, you can see the app running very smoothly as shown in the above. But if you are facing any problem or you have any quires, please feel free to ask it from below comment section.
Let's start the development of this sample with the help of following steps:
Creating a new Project
1. Create a new project from File ⇒ New Flutter Project with your development IDE.
2. Open pubspec.yaml file and add following dependancies
http: ^0.12.0
transparent_image: ^0.1.0
3. After that open main.dart file and edit it. As we have set our theme and change debug banner property of Application. This is our main widget of the example. Here, we have created a grid list widget to display a list of search movies and we have created an instance of MovieApi() to get a list of items from the server.
4. In the following file, we have created a method to access our network layer method. As you can see, we using a POST method to push a query to server and parsing response with the help of base model class.
5. Now create basemodel.dart file. As we said, we use this class to create an object from the server response.
6. Now create PODA class to keep fields of a movie.
7. Here, we have our main file of this post that we were talking about. This is a network util class that we can use it anywhere to perform http request in a Flutter Application. In the following class, we have created a method for GET and POST request. You can add other methods of http according to your requirement. In this file, we have created a field BASE_URL. It contains our base address of the server. We can append a method of server function. Like in this example, we use a search method of themoviedb to get a search result. If you going to build a big application. Then you should use this pattern to perform the server task.
If you have followed the article carefully, you can see the app running very smoothly as shown in the above. But if you are facing any problem or you have any quires, please feel free to ask it from below comment section.