Infinite scroll or load more list is the popular replacement of pagination in a mobile application. With the help of pagination, you can display big data in a small piece of data.
Pagination is a common feature in heavy content apps. It breaks down a list of content into equal smaller pieces and loaded one at a time. Such as, in Facebook, Twitter and Instagram, we spend countless hours scrolling. They just don’t seem to end.
In mobility, if you going to make a app that have a ton of content that takes too long to load. From a developer perspective, how would you load all of that content?. It is not possible to make a call for such huge content at one go. We’ll have to request them in parts or pages.
Pagination allows the user to see the latest content with little wait time. As we load the next page by the time users scroll to the bottom, more content is loaded and available. In mobility, we can implement it with two ways:
Let's create a Futter application and implement a load more feature in the list. I'm assuming, you have basice knowladage of Flutter widgets development and you know list view in Flutter.
We'll create following load more widget:
Creating a new Project
1. Create a new project from File ⇒ New Flutter Project with your development IDE.
2. Open main.dart file and edit it. As we have set our theme and change debug banner property of Application.
3. Now create another dart file and give name home.dart. Here, we'll write our user interface widgets and load more logic. We using english_words 3.1.4 to display list content. We have added this in our project dependency. We'll fetch data from this lib when load more event occurs.
4. To track the last item of list, we using ScrollController. We have created a instance of ScrollController and we have set a listener method. It'll check list item and notify us if reach at last item of the list. Afterthat, we calling startLoader method.
5. StartLoader method will start a progress bar widget and call the fetchData method.
6. In the fetchData method, we have set 2 second delay. Actually, it behaves like an API call request. After 2 second, we calling the onResponse method. It'll update the list of item with new items and stop loader.
The final home.dart final look like below.
If you have followed the article carefully, you can see the app running very smoothly as shown in the above. But if you are facing any problem. If still, you have any quires, please feel free to ask it from below comment section and you can ask it on SLACK.
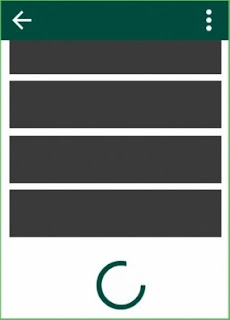
In mobility, if you going to make a app that have a ton of content that takes too long to load. From a developer perspective, how would you load all of that content?. It is not possible to make a call for such huge content at one go. We’ll have to request them in parts or pages.
Pagination allows the user to see the latest content with little wait time. As we load the next page by the time users scroll to the bottom, more content is loaded and available. In mobility, we can implement it with two ways:
- The first option is to add a button to the footer of the view. When pressed it will load more content.
- The second option is to check if the last item is visible and load more content.
Let's create a Futter application and implement a load more feature in the list. I'm assuming, you have basice knowladage of Flutter widgets development and you know list view in Flutter.
We'll create following load more widget:
Creating a new Project
1. Create a new project from File ⇒ New Flutter Project with your development IDE.
2. Open main.dart file and edit it. As we have set our theme and change debug banner property of Application.
3. Now create another dart file and give name home.dart. Here, we'll write our user interface widgets and load more logic. We using english_words 3.1.4 to display list content. We have added this in our project dependency. We'll fetch data from this lib when load more event occurs.
4. To track the last item of list, we using ScrollController. We have created a instance of ScrollController and we have set a listener method. It'll check list item and notify us if reach at last item of the list. Afterthat, we calling startLoader method.
5. StartLoader method will start a progress bar widget and call the fetchData method.
6. In the fetchData method, we have set 2 second delay. Actually, it behaves like an API call request. After 2 second, we calling the onResponse method. It'll update the list of item with new items and stop loader.
The final home.dart final look like below.
If you have followed the article carefully, you can see the app running very smoothly as shown in the above. But if you are facing any problem. If still, you have any quires, please feel free to ask it from below comment section and you can ask it on SLACK.