Crashlytics is a crash and issue reporting service provided by Fabric. It helps developers to build, deploy, and maintain high-quality apps. Fabric will alert you to new issues in real-time by mail and you can find the same issue in the project. You can learn about new issues and fix it.
There is also an opportunity to proactively reach out to users impacted by ongoing issues. fatal and non-fatal issues happening in your app and their complete information with device, orientation, carrier etc. It provides various simple APIs to reports crashes and also stores user information & other details related to crash.
In this tutorial, we will learn how to integrate Crashlytics and track fatal and non-fatal issue in Android application.
Let's start it with registration on Fabric.
1. Register on Fabric
Before starting to integrate, you’ll need to sign up for a free Fabric account. After creating an account, it will send you a confirmation mail. You need to confirm account creation by clicking on the confirmation link received in your inbox. Once, confirmation is complete, you will be redirected to Install Fabric screen and will be asked to choose your IDE.
2. Integrate Fabric Plugin
To use Crashlytics in your project, you need to integrate Fabric plugin in Android Studio as this is the easiest way to integrate Crashlytics kit.
After installing it, restart Android Studio. Now, will see Fabric plugin right side of Android studio.
3. Create a new project in Android Studio.
4. Next step is to integrate Crashlytics in App. Click on the Fabric plugin icon in the toolbar of Android Studio and log in using your credentials.
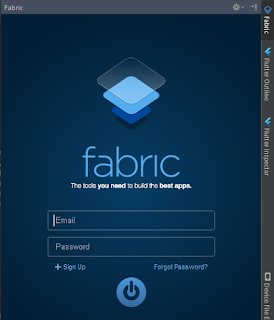
Once logged it will show organizations added in your account. Select an organization for your app and click on Next button.
5. Click on the Next button will take you to the list of all the kits available in Fabric plugin. Select Crashlytics from the list and it will take you to Install screen. Click on the Install button to install Crashlytics kit in your app and accept terms and condition.
During the installation process, Fabric plugin will show you all the modification needed to be done to integrate Crashlytics kit and ask you to confirm it. To confirm, press on next button and plugin will start modifying app’s code. When all modifications are complete then it will ask you to run your application to complete the integration.
6. Now, you will see the whole project is modified by the Fabric.
Fabric will add your API key to the Manifest.xml file:
The fabric may force you to create an application file for put Fabric.with(this, new Crashlytics());. You can add it in MainActivity.java manually as well.
Once integration is complete, Crashlytics will start tracking all the crashes happening in the app without any extra code.
Handle Caught Exceptions.
In addition to automatically reporting your app’s crashes, Crashlytics for Android can also log caught exceptions from app’s catch blocks. To log caught exceptions, add Crashlytics.logException() method to your catch block and pass an exception as an argument.
All caught exceptions logged by Crashlyitcs will appear as a non-fatal issue in Fabric dashboard. Your issue summary will contain all the state information.
So, that's all about Fabric Crashlyitics, But if still, you have any doubt, please feel free to clarify that in the comment section below.
There is also an opportunity to proactively reach out to users impacted by ongoing issues. fatal and non-fatal issues happening in your app and their complete information with device, orientation, carrier etc. It provides various simple APIs to reports crashes and also stores user information & other details related to crash.
In this tutorial, we will learn how to integrate Crashlytics and track fatal and non-fatal issue in Android application.
Let's start it with registration on Fabric.
1. Register on Fabric
Before starting to integrate, you’ll need to sign up for a free Fabric account. After creating an account, it will send you a confirmation mail. You need to confirm account creation by clicking on the confirmation link received in your inbox. Once, confirmation is complete, you will be redirected to Install Fabric screen and will be asked to choose your IDE.
2. Integrate Fabric Plugin
To use Crashlytics in your project, you need to integrate Fabric plugin in Android Studio as this is the easiest way to integrate Crashlytics kit.
After installing it, restart Android Studio. Now, will see Fabric plugin right side of Android studio.
3. Create a new project in Android Studio.
4. Next step is to integrate Crashlytics in App. Click on the Fabric plugin icon in the toolbar of Android Studio and log in using your credentials.
Once logged it will show organizations added in your account. Select an organization for your app and click on Next button.
5. Click on the Next button will take you to the list of all the kits available in Fabric plugin. Select Crashlytics from the list and it will take you to Install screen. Click on the Install button to install Crashlytics kit in your app and accept terms and condition.
During the installation process, Fabric plugin will show you all the modification needed to be done to integrate Crashlytics kit and ask you to confirm it. To confirm, press on next button and plugin will start modifying app’s code. When all modifications are complete then it will ask you to run your application to complete the integration.
6. Now, you will see the whole project is modified by the Fabric.
Fabric will add your API key to the Manifest.xml file:
The fabric may force you to create an application file for put Fabric.with(this, new Crashlytics());. You can add it in MainActivity.java manually as well.
Once integration is complete, Crashlytics will start tracking all the crashes happening in the app without any extra code.
Handle Caught Exceptions.
In addition to automatically reporting your app’s crashes, Crashlytics for Android can also log caught exceptions from app’s catch blocks. To log caught exceptions, add Crashlytics.logException() method to your catch block and pass an exception as an argument.
All caught exceptions logged by Crashlyitcs will appear as a non-fatal issue in Fabric dashboard. Your issue summary will contain all the state information.
So, that's all about Fabric Crashlyitics, But if still, you have any doubt, please feel free to clarify that in the comment section below.